Test Elementor Popups
This guide explains how to use jQuery to trigger an Elementor popup based on different user interactions. We’ll cover how to test between
- Triggering a popup when the user scrolls 50% down the page.
- Triggering a popup after a 20-second delay from page load.
These variations are determined by classes added to the <body>
element by AB split test.
Prerequisites
- jQuery: Some experience with adding / editing JavaScript or jQuery.
- Elementor Pro: The script uses Elementor Pro’s popup module.
- Elementor Popup ID: You should have an Elementor popup created with a specific ID. You can use different popup ID’s if you want to test between popups
- AB Split Test: Installed and activated. Create a new CSS Test
- This example has 2 variations, but you can increase the number of CSS test variations and update the code
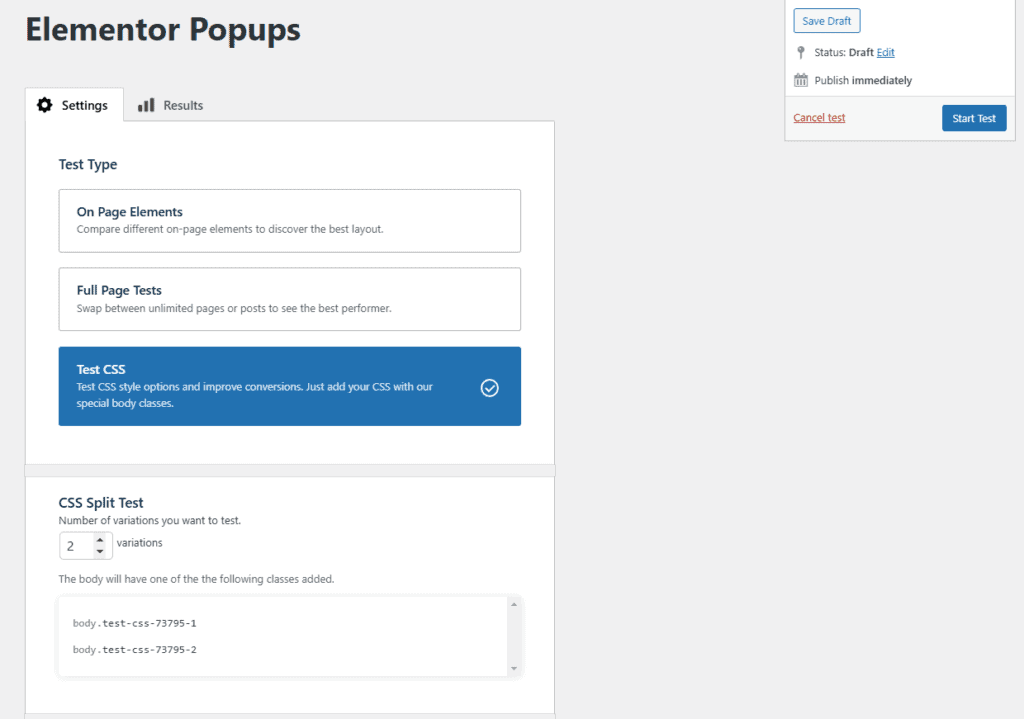
Code Snippet
Add this wherever you add JavaScript code. You need this snippet on every frontend page.
jQuery(document).ready(function() {
jQuery('body').bind('ab-test-setup-complete', function() {
var testId = 73795; // Your test ID
// Check if body has specific class and run corresponding function
if (jQuery('body').hasClass('test-css-' + testId + '-1')) {
scroll50Function();
} else if (jQuery('body').hasClass('test-css-' + testId + '-2')) {
wait20sFunction();
}
});
});
// Define the functions
function scroll50Function() {
// Function to trigger popup at 50% scroll
console.log('Variation: Scroll 50% to trigger popup.');
jQuery(window).on('scroll.scrollPopup', function() {
var scrollTop = jQuery(window).scrollTop();
var docHeight = jQuery(document).height();
var winHeight = jQuery(window).height();
var scrollPercent = scrollTop / (docHeight - winHeight);
if (scrollPercent >= 0.5) {
// Unbind the scroll event so this only happens once
jQuery(window).off('scroll.scrollPopup');
// Trigger the Elementor popup
elementorProFrontend.modules.popup.showPopup({ id: 123 }); // Replace 123 with your actual popup ID
console.log('Popup triggered at 50% scroll.');
}
});
}
function wait20sFunction() {
// Function to trigger popup after 20 seconds
console.log('Variation: Wait 20 seconds to trigger popup.');
setTimeout(function() {
// Trigger the Elementor popup
elementorProFrontend.modules.popup.showPopup({ id: 123 }); // Replace 123 with your actual popup ID
console.log('Popup triggered after 20 seconds.');
}, 20000); // 20000 milliseconds = 20 seconds
}
How It Works
1. Setting the Test ID
var testId = 73795; // Your test ID
- Purpose: Identifies the test and is used to construct the body class names.
- Action: Replace
73795
with your actual test ID.
2. Checking for Body Classes
Purpose: Determines which variation function to execute based on the body class.
- Mechanism:
- Checks if the
<body>
element has a class liketest-css-6833-1
ortest-css-6833-2
. - Executes
scroll50Function()
if the class istest-css-6833-1
. - Executes
wait20sFunction()
if the class istest-css-6833-2
.
- Checks if the
3. The scroll50Function
function scroll50Function() {
// Function to trigger popup at 50% scroll
console.log('Variation: Scroll 50% to trigger popup.');
jQuery(window).on('scroll.scrollPopup', function() {
var scrollTop = jQuery(window).scrollTop();
var docHeight = jQuery(document).height();
var winHeight = jQuery(window).height();
var scrollPercent = scrollTop / (docHeight - winHeight);
if (scrollPercent >= 0.5) {
// Unbind the scroll event so this only happens once
jQuery(window).off('scroll.scrollPopup');
// Trigger the Elementor popup
elementorProFrontend.modules.popup.showPopup({ id: 123 }); // Replace 123 with your actual popup ID
console.log('Popup triggered at 50% scroll.');
}
});
}
- Purpose: Triggers the popup when the user has scrolled 50% down the page.
- How It Works:
- Attaches a scroll event listener to the window.
- Calculates the user’s scroll position as a percentage of total page height.
- Checks if the scroll percentage is 50% or more.
- Unbinds the scroll event to prevent multiple triggers.
- Triggers the Elementor popup.
4. The wait20sFunction
function wait20sFunction() {
// Function to trigger popup after 20 seconds
console.log('Variation: Wait 20 seconds to trigger popup.');
setTimeout(function() {
// Trigger the Elementor popup
elementorProFrontend.modules.popup.showPopup({ id: 123 }); // Replace 123 with your actual popup ID
console.log('Popup triggered after 20 seconds.');
}, 20000); // 20000 milliseconds = 20 seconds
}
- Purpose: Triggers the popup 20 seconds after the page has loaded.
- How It Works:
- Uses
setTimeout
to delay the popup trigger by 20,000 milliseconds (20 seconds). - Triggers the Elementor popup after the delay.
- Uses
Troubleshooting
- Popup Doesn’t Appear:
- Check the Popup ID: Ensure you’ve replaced
123
with the correct popup ID. - Verify Elementor Conditions: Make sure the popup isn’t restricted by conditions set within Elementor.
- Console Errors: Open the browser console to check for JavaScript errors.
- Check the Popup ID: Ensure you’ve replaced
- Function Not Executing:
- Body Class: Confirm that the
<body>
element has the correct test class that you have defined in the javascript. - Script Load Order: Ensure that jQuery and Elementor Pro scripts are loaded before this script.
- Body Class: Confirm that the
- Scroll Percentage Not Calculating Correctly:
- Page Height Issues: If your page has dynamic content or lazy-loaded elements, the document height might change.
- Adjust Threshold: Modify the scroll percentage threshold if needed.