Split Test Featured Images Using CSS Test Mode
Introduction
It’s easy to test featured images on your WordPress pages or posts using the CSS test mode. By applying a custom JavaScript snippet, you can dynamically change the featured image based on the test variation assigned to the user. This article walks you through how to implement this for a specific post or page.
Steps to Implement
- Get the page ID that the featured image is displayed on:
- Go to the page with the featured image that you want to swap.
- Inspect the source, and see the body will have postid-1234 or page-id-1234
- the ‘1234’ is your page or post ID
- Go to the page with the featured image that you want to swap.
- Create a new CSS Split Test
- Give it a name, choose your goals and start the test.
- Take note of the Test ID, shown under CSS Split Test
- If your split test classes are .test-css-1729-1
- 1729 is your test ID
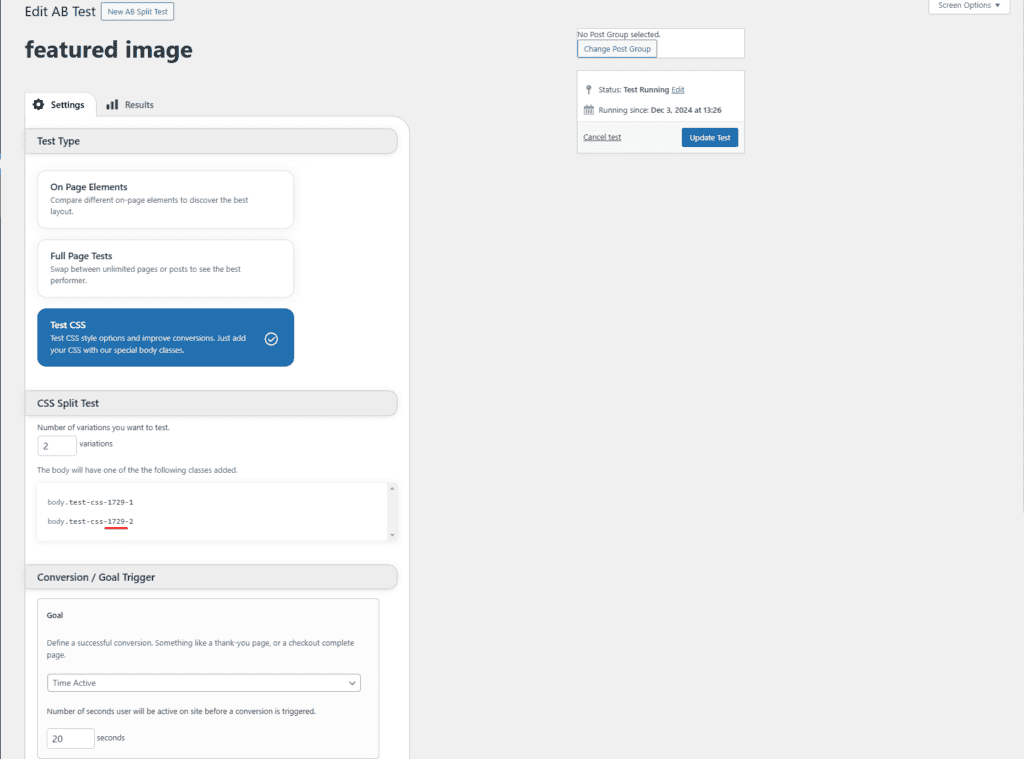
- Upload your alternate featured image:
- Add your other image to the medial library
- Copy the File URL link to the image
Now we add our values to the snippet below and then add the snippet to our website.
Custom Script for Testing Featured Images
Below is the custom JavaScript code that needs to be added to your page or website to test featured images:
jQuery(document).ready(function () {
const pageId = 1234; // Target post/page ID to test on
const testId = 1729; // Your CSS test ID
const alternateImageUrl = 'https://placehold.co/600x400'; // Alternate featured image URL for testing
// Listen for the setup event only if the body class matches the target page
jQuery(`body.postid-${pageId}, body.page-id-${pageId}`).on('ab-test-setup-complete', function () {
const testDetails = JSON.parse(abstGetCookie(`btab_${testId}`)); // Retrieve test details for the current user
if (testDetails && testDetails.variation === `test-css-${testId}-2`) {
// Update the image source for the second test variation
jQuery('img.wp-post-image')
.attr('src', alternateImageUrl) // Replace the image source
.removeAttr('srcset'); // Remove the srcset attribute for proper image display
console.log('featured image swapped');
}
});
});
How the Script Works
- Body Class Check: The script first ensures that the test runs only on the specified post/page by checking the body class.
- Event Listener: It listens for the
ab-test-setup-complete
event to ensure the test setup is completed before making changes. - Cookie Retrieval: The script retrieves the user’s test variation from the cookie using the
abstGetCookie
function. - Variation Check: If the user is assigned to variation 2, the script updates the featured image’s
src
attribute and removes thesrcset
attribute to prevent conflicts.
Important Notes
- Alternate Image Preparation: Ensure your alternate image is optimized for web and is the correct size to avoid layout issues.
- Make sure your existing featured image doesn’t have lazy loading or another feature that may change the image source.