Server Side Events
Introduction
Server-side event tracking in AB Split Test allows you to log experiment activity directly on your server, improving tracking accuracy and supporting Google Tag Manager (GTM) events. This guide explains how to enable advanced tracking, retrieve the user’s Advanced ID, and send events using both PHP and AJAX.
1. Enabling Server-Side Tracking
- Go to: WordPress Admin > Settings > AB Split Test
- Enable “Advanced Tracking” (as shown in the screenshot).
- Set the UUID validity (the number of days the user identifier should persist).
- Click Save Changes.
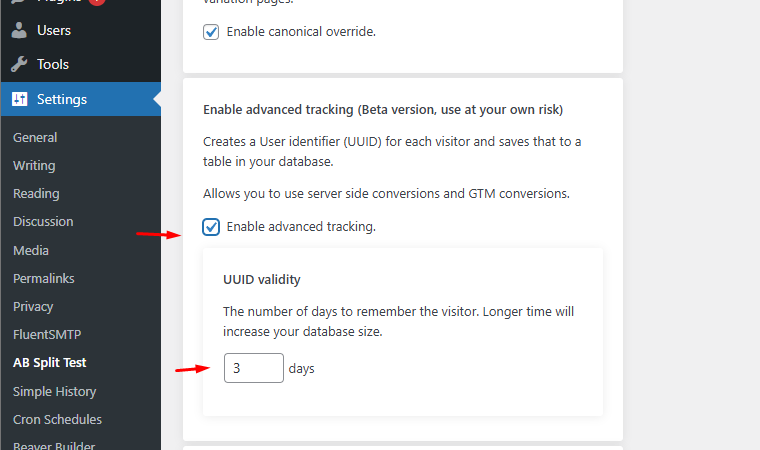
Once enabled, a unique user identifier (UUID) is assigned to each visitor and stored in your database for the number of days you choose with UUID Validity. This ID can be used for tracking and server-side event logging.
2. Retrieving the User’s Advanced ID
Retrieve it using JavaScript:
const advancedId = localStorage.getItem('ab-advanced-id');
console.log("Advanced ID: ", advancedId);
Retrieve it with PHP
<?php
$advancedId = $_COOKIE['ab-advanced-id'];
Alternatively, we also send this identifier via Google Tag Manager (GTM), where it can be used in event tracking.
3. Creating a Server-Side Event Using PHP
Example PHP Function to Log a Conversion
You can log a conversion using the bt_log_experiment_activity
hook available inside WordPress.
/**
* Log experiment activity manually via PHP
*
* @param int $bt_eid The experiment ID
* @param string $bt_variation The variation ID
* @param string $bt_type The type of activity (visit|conversion|goal)
* @param bool $from_api Whether the request is coming from a js navigator.beacon() call or the AB Test admin page
* @param string $bt_location The location of the activity
* @param int $abConversionValue The conversion value (optional)
* @param string $fingerprintID The user's Fingerprint ID or false
* @param string $size The device type (mobile|tablet|desktop)
* @param string $advancedId The user's advanced ID
*/
log_experiment_activity($bt_eid, $bt_variation, $bt_type, $from_api, $bt_location, $abConversionValue, $fingerprintID, $size, $advancedId);
Example PHP Request to Log a Conversion:
//..other code that triggers the event..
$test_id = 1729; // your test you want to convert
$event_type = "conversion";
$variation = null; //already set during visit
$location = "my_function_trigger";
$conversion_value = 1; // Example: revenue from an order in whole dollars/euros etc or leave as 1
$advancedId = false; // Or use $_COOKIE['ab-advanced-id'];
do_action('bt_log_experiment_activity', $test_id, $variation, $event_type, true, $location, $conversion_value, false, false, $advancedId); // send conversion event
// something else
4. Sending a Conversion Event from an external domain via AJAX
If you want to send conversions using AJAX, add the following WordPress hooks to handle logged-in and guest users:
Example JavaScript AJAX Request to Log a Conversion:
const testId = 1729;
const variation = 'ab-test-variation';
const eventType = 'conversion';
const advancedId = localStorage.getItem('ab-advanced-id');
const conversionValue = 1; // Example: revenue from an order
const ajaxurl = 'https://mywebsite.com/wp-admin/wp-ajax.php';
jQuery.ajax({
url: ajaxurl, // WordPress AJAX handler
type: 'POST',
data: {
action: 'abst_event',
bt_eid: testId,
bt_variation: variation,
bt_type: eventType,
bt_location: window.location.href,
abConversionValue: conversionValue,
advancedId: advancedId
},
success: function(response) {
console.log('Conversion event logged:', response);
},
error: function(error) {
console.error('Error logging event:', error);
}
});
5. Logging Events via Google Tag Manager (GTM)
AB Split Test automatically sends the Advanced ID and test ID to Google Tag Manager (GTM), allowing you to use it in GTM triggers. You can configure GTM to collect this data, then use it to trigger conversions at a later date inside tag manager.